How Developers Can Improve Their Debugging Competencies By Gustavo Woltmann
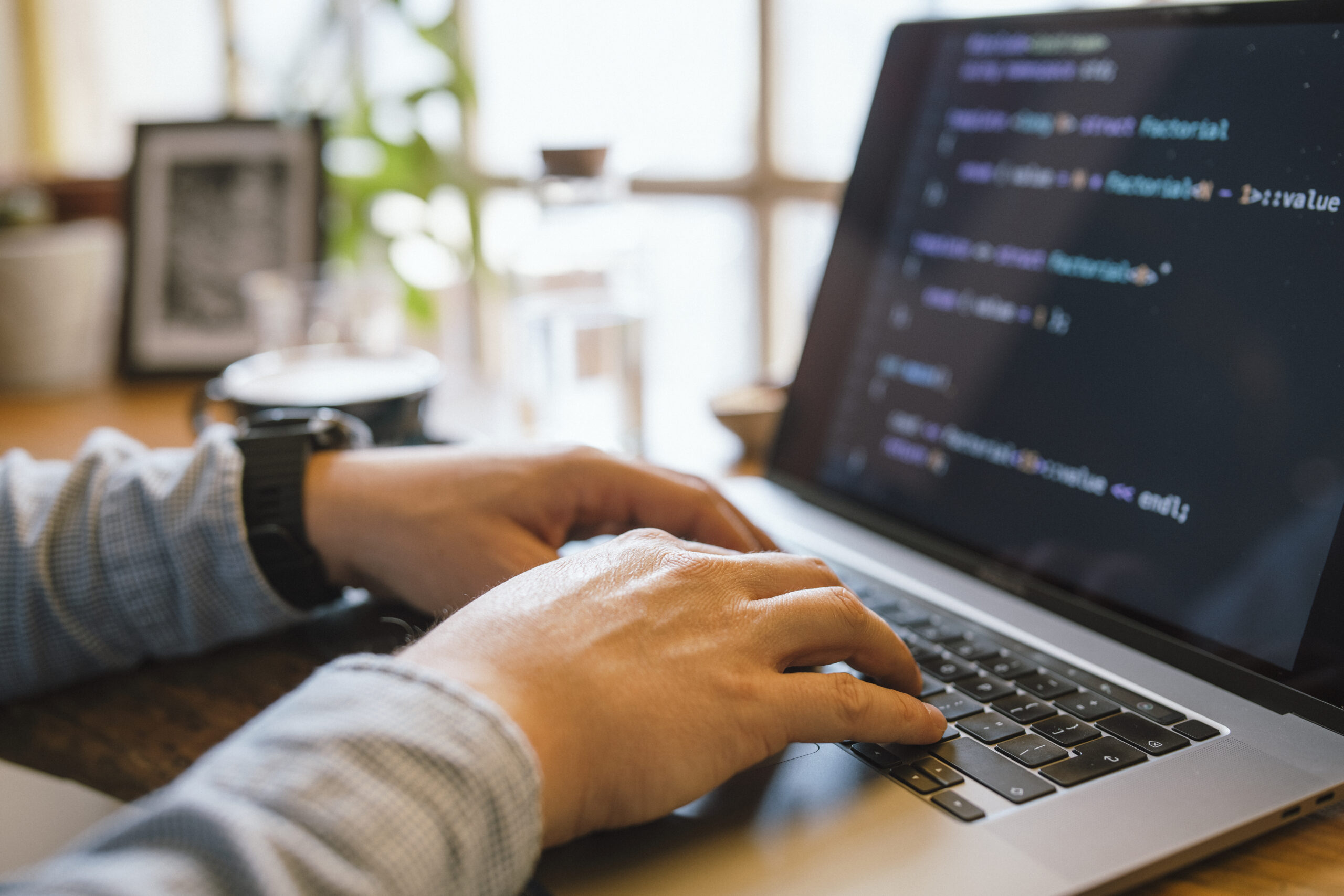
Debugging is Probably the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve troubles successfully. Irrespective of whether you are a rookie or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of fastest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though composing code is 1 part of enhancement, figuring out the way to interact with it correctly during execution is Similarly vital. Present day improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Just take, for instance, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment let you established breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When applied accurately, they let you notice precisely how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, watch network requests, look at real-time effectiveness metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can transform irritating UI difficulties into workable tasks.
For backend or technique-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory management. Studying these instruments can have a steeper Studying curve but pays off when debugging effectiveness problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with Edition Regulate units like Git to understand code background, uncover the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies heading further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when difficulties occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may shell out fixing the particular difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the condition. In advance of leaping in to the code or creating guesses, developers have to have to produce a regular setting or situation exactly where the bug reliably seems. Without the need of reproducibility, repairing a bug turns into a video game of possibility, usually leading to squandered time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What steps brought about the issue? Which environment was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater depth you have, the a lot easier it will become to isolate the exact disorders beneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood setting. This could signify inputting the identical details, simulating comparable person interactions, or mimicking method states. If The problem seems intermittently, think about producing automated assessments that replicate the sting circumstances or point out transitions involved. These exams don't just assist expose the challenge but also avoid regressions Down the road.
Occasionally, the issue could possibly be ecosystem-particular — it would materialize only on particular running units, browsers, or below unique configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t merely a step — it’s a way of thinking. It requires patience, observation, along with a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to fixing it. Having a reproducible situation, You need to use your debugging equipment additional proficiently, exam opportunity fixes safely, and communicate much more Obviously along with your staff or buyers. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Study and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then looking at them as annoying interruptions, developers ought to discover to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it happened, and in some cases even why it took place — if you know how to interpret them.
Start by looking at the concept cautiously As well as in entire. Numerous developers, particularly when below time tension, look at the very first line and straight away start off creating assumptions. But further while in the error stack or logs may perhaps lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and understand them 1st.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and point you toward the liable code.
It’s also useful to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging procedure.
Some glitches are imprecise or generic, and in People conditions, it’s essential to examine the context wherein the error occurred. Check out similar log entries, input values, and recent alterations from the codebase.
Don’t overlook compiler or linter warnings either. These typically precede larger sized problems and provide hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A good logging technique starts with knowing what to log and at what level. Common logging levels consist of DEBUG, INFO, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic facts through progress, Data for basic activities (like productive begin-ups), Alert for opportunity difficulties that don’t split the application, Mistake for genuine difficulties, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Target crucial events, point out adjustments, enter/output values, and significant choice points in the code.
Format your log messages Evidently and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically valuable in creation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging technique, you can reduce the time it's going to take to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex task—it's a kind of investigation. To proficiently identify and correct bugs, builders will have to approach the process similar to a detective resolving a mystery. This attitude will help stop working elaborate issues into manageable elements and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you may without the need of leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, type hypotheses. Inquire your self: What might be causing this actions? Have any variations not long ago been designed on the codebase? Has this situation transpired just before beneath related conditions? The aim is usually to narrow down possibilities and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the challenge in a very controlled surroundings. Should you suspect a particular operate or part, isolate it and confirm if the issue persists. Like a detective conducting interviews, inquire your code concerns and let the results guide you nearer to the truth.
Spend close interest to smaller aspects. Bugs normally conceal in the the very least anticipated places—just like a missing semicolon, an off-by-one error, or perhaps a race issue. Be thorough and affected person, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes may conceal the true problem, only for it to resurface later on.
Last of all, keep notes on Whatever you tried using and discovered. Just as detectives log their investigations, documenting your debugging approach can help you save time for long term troubles and enable others realize your reasoning.
By wondering similar to a detective, builders can sharpen their analytical techniques, solution issues methodically, and come to be more effective at uncovering concealed issues in sophisticated techniques.
Compose Tests
Writing exams is one of the most effective ways to enhance your debugging expertise and All round advancement efficiency. Tests not only aid catch bugs early but in addition serve as a safety net that gives you self-assurance when generating modifications to the codebase. A nicely-examined software is much easier to debug as it allows you to pinpoint just exactly where and when a challenge occurs.
Get started with unit assessments, which give attention to individual features or modules. These little, isolated exams can quickly reveal whether a certain piece of logic is Performing as expected. Any time a check fails, you quickly know wherever to glimpse, noticeably reducing some time used debugging. Device checks are especially useful for catching regression bugs—problems that reappear following previously being fixed.
Up coming, integrate integration tests and close-to-close assessments into your workflow. These support be certain that different elements of your software get the job done jointly easily. They’re especially helpful for catching bugs that occur in complicated systems with many elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Consider critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge cases. This standard of knowing The natural way qualified prospects to raised code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your exam pass when The problem is solved. This approach ensures that the exact same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable process—assisting you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—observing your monitor for several hours, trying Answer right after Remedy. But Among the most underrated debugging applications is solely stepping absent. Having breaks helps you reset your thoughts, decrease disappointment, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer way of thinking. You could suddenly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, enhances your perspective, and aids you check here steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to increase for a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can instruct you something useful when you take the time to reflect and evaluate what went Improper.
Start off by inquiring you a couple of crucial inquiries when the bug is solved: What prompted it? Why did it go unnoticed? Could it are actually caught earlier with better practices like unit testing, code reviews, or logging? The answers often reveal blind spots in your workflow or comprehending and enable you to Construct more powerful coding behavior relocating forward.
Documenting bugs can be a superb routine. Preserve a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In group environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast expertise-sharing session, assisting Many others stay away from the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, a few of the finest developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you correct provides a fresh layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Whatever you do.